This question is taken from book .NET interview questions and answers published by Bpb publication and written by Shivprasad koirala.
Many time classes have contained (aggregated or composed) collections. For example in the below code you can see we have a customer class which has an address collection.
public class Customer
{
private List<Address> Addresses = new List<Address>();
}
Now let’s say we would like to like fetch the addresses collection by “Pincode” and “PhoneNumber”. So the logical step would be that you would go and create two overloaded functions one which fetches by using “PhoneNumber” and the other by “PinCode”. You can see in the below code we have two functions defined.
public Address getAddress(int PinCode)
{
foreach (Address o in Addresses)
{
if (o.Pincode == PinCode)
{
return o;
}
}
return null;
}
public Address getAddress(string PhoneNumber)
{
foreach (Address o in Addresses)
{
if (o.MobileNumber == PhoneNumber)
{
return o;
}
}
return null;
}
Now on the client side your code would look something as shown below.
Customer Customers = new Customer();
Customers.getAddress(1001);
Customers.getAddress(“9090″);
Now the above code is great but how about simplifying things further. How about something more simple as shown in the below code. In other words we want to INDEXED the class itself.
Customer Customers = new Customer();
Address o = Customers[10001];
o = Customers["4320948"];
You can see the below image where we can have two overloaded indexers “PinCode” and “PhoneNumber”.
This can be achieved by using an indexer. There are two things we need to remember about indexer:-
“Indexer” is defined by using “this” keyword.
“Indexer” is a property so we need to define set and get for the same.
Below is the code implementation for indexer. You can see we have used “this” keyword with two overloaded function one which will fetch us address by using the “phonenumber” and the other by using “pincode”.
public class Customer
{
private List<Address> Addresses = new List<Address>();
public Address this[int PinCode]
{
{
foreach (Address o in Addresses)
{
if (o.Pincode == PinCode)
{
return o;
}
}
return null;
}
}
public Address this[string PhoneNumber]
{
{
foreach (Address o in Addresses)
{
if (o.MobileNumber == PhoneNumber)
{
return o;
}
}
return null;
}
}
}
Once you put the above “indexer” code you should be able to access the address collection from the customer object using an indexer “[]” as shown in the below figure.
Summarizing in one sentence: – Indexers helps to access contained collection with in a class using a simplified interface. It’s a syntactic sugar.
You can see csharp ( c# / .NET ) GAC interview questions and answers from www.questpond.com
More .NET and c# interview question videos:-
C# ( Csharp) interview question :- What are checked and unchecked keyword in .NET ?
c# exception handling interview questions with answer :- Difference between Throw and Throw ex ?
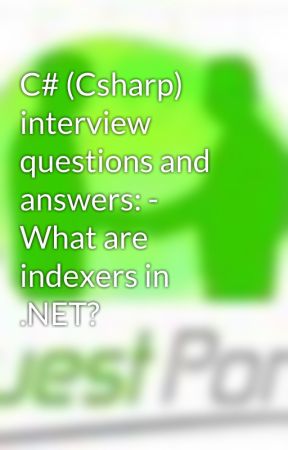